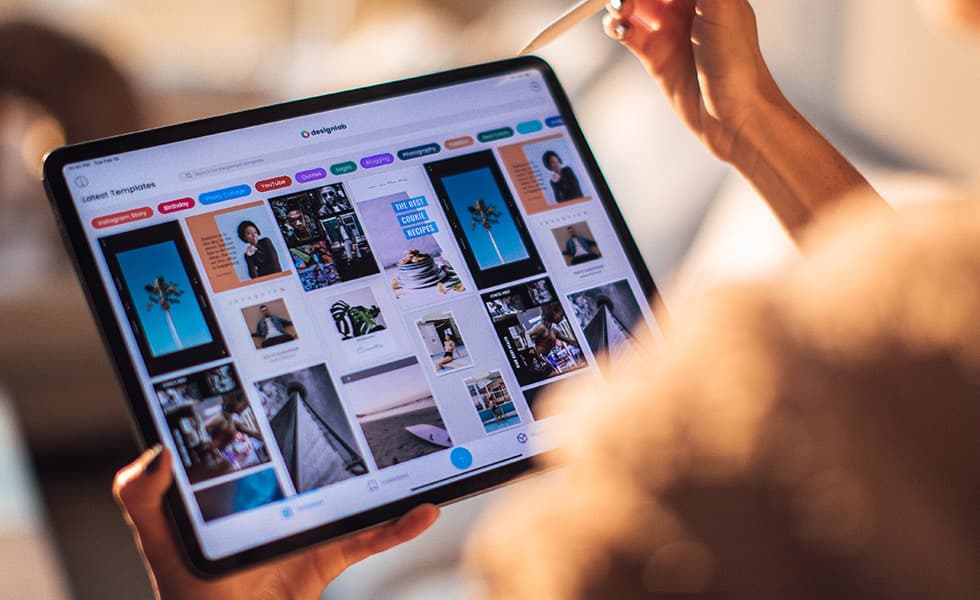
Understanding React Hooks: A Comprehensive Guide
React Hooks revolutionized the way we manage state and side effects in React components. If you're looking to grasp the ins and outs of this powerful feature, you're in the right place. This guide will take you through everything you need to know about React Hooks, from the basics to advanced usage, helping you write cleaner, more efficient, and maintainable React code.
What are React Hooks:
Begin by understanding the motivation behind React Hooks and how they simplify state management and side effects in functional components.
Basic Hooks:
Dive into the fundamental hooks for managing state and handling side effects. Learn how to bring lifecycle functionalities into functional components.
State Management with useState:
Explore how to create and manage component state using the "useState" hook. Discover techniques to handle different types of data and manage complex state structures.
Effect Side Effects with useEffect:
Master the "useEffect" hook, which enables you to manage side effects like data fetching, DOM manipulation, and more. Understand dependencies, cleanup, and the order of execution.
Custom Hooks:
Uncover the magic of custom hooks. Learn how to create your own reusable hooks to encapsulate logic and stateful behavior across different components.
Context with useContext:
Delve into the "useContext" hook for efficient context management. Understand how to share data and functions across components without prop drilling.
Optimizing Performance:
Explore strategies to optimize performance using React Hooks. Learn about memoization, dependency arrays, and techniques to avoid unnecessary renders.
Time-Traveling with useRef:
Discover the "useRef" hook, which is not just for references. Explore how it can be used for managing persistent values and interacting with DOM elements.
Combining Hooks:
Learn how to effectively combine multiple hooks within a component to achieve complex functionality without compromising readability.
Async Operations with useEffect:
Dive into handling asynchronous operations with the "useEffect" hook. Manage data fetching, API calls, and error handling with ease.
Global State with Redux and useReducer:
Understand how "useReducer" can be used for managing global state and how it compares to state management libraries like Redux.
Testing and Debugging Hooks:
Explore techniques for testing and debugging components that utilize React Hooks. Discover tools and practices to ensure your hooks work as expected.
Continual Learning:
React Hooks are a dynamic area of development. Learn how to stay up-to-date with the latest advancements and best practices in the world of React.
By the end of this comprehensive guide, you'll be well-equipped to leverage the power of React Hooks to create more efficient, modular, and maintainable React applications. Get ready to level up your React skills and build modern UIs that are easier to understand and maintain. Happy hooking! ⚛️